Table of Contents
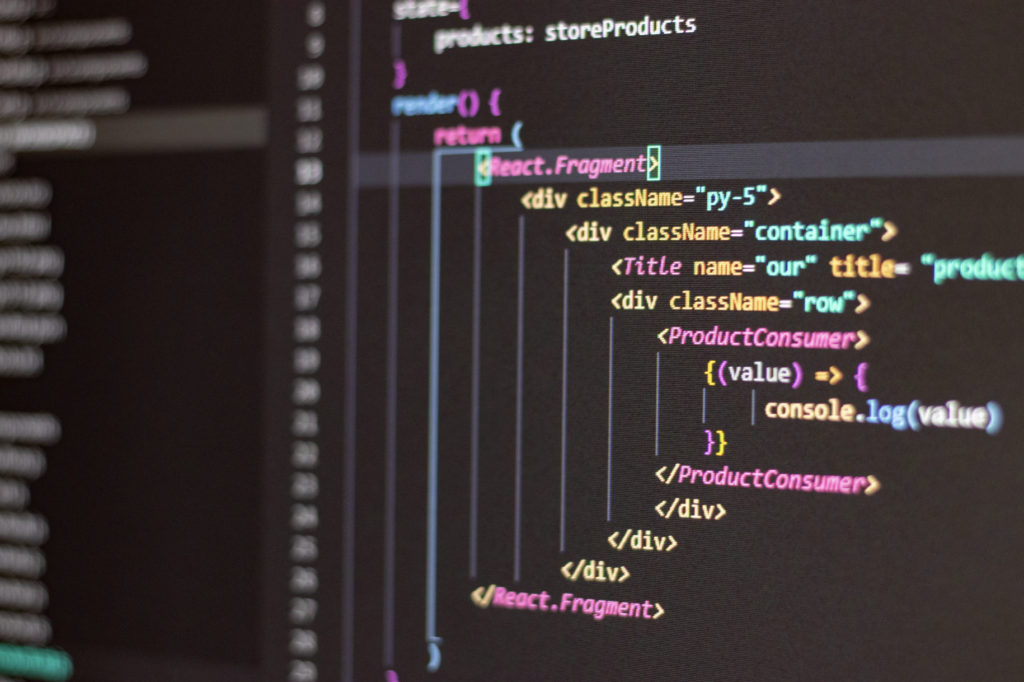
Easy Level Questions:
Explain each of the following terms (algorithm design(:
a. symmetric encryption
b. asymmetric encryption
c. hashing algorithm
d. cypher text
e. encryption algorithm
f. key distribution problem
a uses a secret key which when applied to a message makes the message
unreadable without this key
b form of encryption that uses public keys and private keys
c takes a message or key and translates it into a string of characters
shown in hex notation
d output following plain text being put through an encryption algorithm
e converts messages using an encryption key into
unreadable/meaningless text
f in symmetric encryption, where encryption key can be intercepted
during transmission putting security of message at risk
Give two disadvantages of writing a program in a high-level language.
– A program written in a high-level language takes a longer time to complete the same task than a program written in a low-level language.
– A program written in a high-level language takes up more space in memory than a program written in a low-level language to perform the same task.
Give two disadvantages of writing a program in a low-level language.
– Programs written in a low-level language are more difficult for programmers to understand than programs written in a high-level
language.
– Programs written in a low-level language take longer for programmers to debug than programs written in a high-level language.
What kind of language is this? 1 12, 4 13, 0 1A
– Machine code (hexadecimal)
What is the build automation?
– Ide tool. These tools save time by automatically doing the processes that would otherwise be done by hand. These could include testing or compiling. These tools are extremely useful when a program has many thousands of lines of code.
What is libraries?
– IDE tool. These provide functions that are not included in the core part of the programming language. These functions can be imported and used at the start of the program code. For example, in Python the Turtle Graphics library provides access to some simple drawing and graphics tools.
What is auto documentation?
– IDE tool. This explains the function and purpose of the code, eg by noting the modules and variables used, and its expected behaviour, and collates this into a text file that can be used by other developers to understand how and why the code was created.
What is translators?
– IDE tool. This compiles or interprets the code.
What are the different IDE tools?
– translator
– auto documentation
– libraries
– build automation
What is syntax checks?
– Code editor features. This recognizes incorrect use of syntax and highlights any errors.
What is bracket matching?
– Code editor feature. This is used for languages that use pairs of brackets to mark out blocks of code. It allows the code to be read and understood more quickly. If you forget to close a bracket while writing, colored sections may help you to detect missing brackets.
What is auto-completion?
– Code editor feature. This is designed to save time while writing code. As you start to type the first part of a function, it suggests or completes the function and any arguments or variables.
What are the features of code editor features?
– auto completion
– bracket matching
– syntax checks
Explain what is meant by IDEs having runtime environment?
– This means that you can execute the program one step at a time.
– This is useful to test that the code is working line by line before creating the final complete program.
Medium Level Questions:
Define code editor?
– The environment where the user can write code is called the shell.
– The code editor is a text edit area that allows developers to write, edit and save a document of code.
Explain what is meant by IDE?
– An integrated development environment (IDE) is an application used to create software.
– An IDE can often support different languages.
– IDEs have a number of different tools and functions that assist a developer in the creation of software.
What is runtime errors?
– RUNTIME ERRORS are errors which will cause the program or computer to crash even if there appears to be nothing wrong with the program code. Running out of memory will often cause a runtime error. This could be because instructions have been written in the wrong order.
What is logic/semantic error?
– A LOGIC/SEMANTIC ERROR is where the program doesn’t do what the programmer wanted it to do. Logic errors are found when a program is being run. These can be found by tracing what the program does and using test data with expected results
Explain a syntax error?
– Is where a program statement doesn’t obey the rules of the programming language.
– A program cannot be translated if it contains syntax errors.
What are the different types of an error?
– Syntax error
– Logic/semantic error
– Runtime errors
How does virtual machine work?
– A system virtual machine is a software application run by the host operating system of a computer which emulates a second operating system.
– This permits the installation and execution of software applications on the virtual machine (VM) as if they were being installed and run on a separate computer.
– For example, it is possible to run a Microsoft Windows Virtual Machine on an Apple Mac – allowing software applications that only work on the Microsoft Windows OS to be run via the VM, which in turn is run on the Mac.
Give examples of virtual machine?
– For example, the Java Virtual Machine allows Java bytecode produced on a Microsoft Windows OS to run on a Unix OS without any changes.
Define virtual machine?
– A process virtual machine is a programming environment that allows a program written for one type of machine to run on other types of machine without any changes being necessary.
Give summary of assembler?
– Translates a low-level language program into machine code
– An executable file of machine code is produced
– One low level language statement is usually translated into one machine code instruction
– Assembled programs can be used without the assembler
– An assembled program is usually distributed for general use
Give summary of interpreter?
– Executes a high-level language program, one statement at a time
– No executable file of machine code is produced
– One high-level language program statement may require several machine code instructions to be executed
– Interpreted programs cannot be used without the interpreter
– An interpreter is often used when a program is being developed
Give summary of compiler?
– Translates a high-level language program into machine code
– An executable file of machine code is produced
– One high-level language statement can be translated into several machine code instructions
– Compiled programs are used without the compiler
– A compiled program is usually distributed for general use
Explain what is meant by assemblers?
– A computer program that translates a program written in an assembly language into machine code so that it can be directly used by a computer to perform a required task.
– Once a program is assembled the machine code can be used again and again to perform the same task without re-assembly.
What are the uses of macro?
– Macros can be used to make tasks less repetitive by representing a complicated sequence of keystrokes, mouse movements, commands, or other types of input.
– Macro is a single line abbreviation for group of instructions.
– With the help of macro, programmer can define a single “instruction” to represent block of code.
Define macro?
– A macro (which stands for “macroinstruction”) is a programmable pattern which translates a certain sequence of input into a preset sequence of output.
Mastery Level Questions:
What is the use of loader?
– It calculates the size of a program (instructions and data) and create memory space for it. It initializes various registers to initiate execution.
Define loader?
– Loader is a part of operating system and is responsible for loading executable files into memory and execute them.
What is the major task of linker?
– The major task of a linker is to search and locate referenced module/routines in a program and to determine the memory location where these codes will be loaded making the program instruction to have absolute reference.
Define linker?
– In computer science, a linker is a computer program that takes one or more object files generated by a compiler and combines them into one, executable program.
What are the disadvantages of a compiler over an interpreter?
– More memory is required during compilation because the machine code program is held in main memory as well as the high level language program.
– Errors take longer to find as the whlole program need to be edited and recompiled after every error.
– Programs take longer to develop as the program needs to be restarted from the beginning when an error is found rather than continuing from where the error was once it has been corrected.
What are the advantages of a compiler over an interpreter?
– Less memory is required when the program is run because the compiler is not held in main memory just the compiled program.
– Program does not need to be recompiled every time it is used unlike an interpreted program.
– Program executed faster as statements are not re-translated every time.
Define bytecode?
– Bytecode is code which is compiled and can then be interpreted.
Explain Java and C++ using compiler?
– Java and C++ are compiled programming languages. Java is a high-level programming language which is compiled to produce bytecode which is then interpreted by a virtual machine (VM).
How does compiler work?
– Once a program is compiled the machine code can be used again and again to perform the same task without recompilation.
– It can be difficult to test individual lines of compiled code compared to interpreted languages as all bugs are reported after the program has been compiled.
– The machine code is saved and stored separately to the high-level code. Compilation is slow but machine code can be executed quickly.
Define compiler?
– A compiler is a computer program that translates a program written in a high-level language (HLL) into machine code so that it can be directly used by a computer to perform a required task.
How does machine code work?
– It is represented by binary numbers
– Programmers do not usually write in machine code as it is difficult to understand and it can be complicated to manage data manipulation and storage.
Define machine code?
– Machine code also called as object-code.
– Machine code is a low-level code that represent how computer hardware and CPUs understand instructions.
How does stepping work?
– A debugger can also use breakpoints (points in the code where the program can be stopped to see what is happening and check for errors).
– A breakpoint can be created by the programmer to halt the program after a certain number of lines of code.
– In software development, a breakpoint is an intentional stopping or pausing place in a program, put in place for debugging purposes. It is also sometimes simply referred to as a pause.
Define stepping?
– Stepping is a method of debugging which executes the code one line at a time to check for errors.
How does debugging work?
– A debugger program is usually included within the IDE.
– If a section of code gives you different results from what you would expect, try to make that section of code work in isolation.
– You may have to give it ‘dummy’ values to make it work.
Define debugging?
– Debugging is the process of working through the program in a systematic way to eliminate any flaws or glitches.
What is the use of interpreter?
– These are ideal for using within dynamic web applications. They are used for client-side and server-side coding, as they are small programs that are executed within the browser.
Give examples of interpreted languages?
– Interpreted languages include JavaScript, PHP, Python and Ruby.
– Interpreted languages are also called scripting languages.
How does interpreter work?
– Interpreted code will show an error (usually syntax error) as soon as it hits a problem, so it is easier to debug than compiled code.
– An interpreter does not create an independent final set of source code – source code is created each time it runs. Interpreted code is slower to execute than compiled code.
Explain what is meant by interpreter?
– An interpreter translates code from a high level program into machine code, instruction by instruction – the CPU executes each instruction before the interpreter moves on to translate the next instruction.
Explain what is meant by translators?
– Translators is a utility program that translate a program into binary before a computer can use it.
– Programs written by humans (HLL) are easily understood by programmers but computers cannot understand it.
– So the program must be translated into binary instructions, or machine code so that the computer is able to understand it.
– Assembly language also needs translators to be executed.
What does STO mean?
– STO means replace the value of the variable by the value stored in the accumulator.
What does ADD mean?
– ADD means add the value of another variable to the value stored in the accumulator
What does LDA mean?
– LDA means load the value of the variable into the accumulator
What are the 3 terms of assembly languages?
– LDA
– ADD
– STO
Disadvantages of assembly languages?
– Long programs written in such languages cannot be executed on small sized computers.
– It takes lot of time to code or write the program, as it is more complex.
– Difficult to remember the syntax.
– To write programs in assembly language, the internal structure of the microprocessor must be known.
– Assembly language varies by microprocessor type. A program written for a microprocessor may not work on another microprocessor. The program is not independent of the portable platform.
– Programming in assembly language is more difficult and time consuming than highlevel languages.
Advantages of assembly languages?
– Can make use of special hardware or special machine-dependent instructions (e.g. on the specific chip)
– Translated program requires less memory
– Write code that can be executed faster
– Total control over the code
– Can work directly on memory locations
Examples of assembly languages?
– IBM PC DOS, used in IBM personal computer
– Turbo Pascal compiler, used in the Nascom microcomputer
Give examples of mnemonic?
– For example, many menu options are displayed with an underlined character, representing a key to be pressed to access that menu or option.
– The shortcut key for opening the File menu is F, which stands for “file”. You can press Alt + F or Command + F to access the file menu using your keyboard.
Explain what is meant by mnemonic?
– A mnemonic is a visual or auditory aid that helps an individual remember something. In computers, mnemonics are often used to make a shortcut easy to remember.
What are the reasons to use assembly languages?
– to make use of special hardware
– to make use of special machine-dependent instructions
– to write code that doesn’t take up much space in primary memory
– to write code that performs a task very quickly.
Explain what is meant by assembly languages?
– Assembly language is a low-level language written in mnemonics that closely reflects the operations of the CPU.
– Few programmers write programs in an assembly language.
Give examples of low level languages?
– Machine language
– Assembly language
Use of low level languages?
– A low-level language is a programming language that deals with a computer’s hardware components.
– Low-level languages are designed to operate and handle the entire hardware and instructions set architecture of a computer directly.
– Their prime function is to operate, manage and manipulate the computing hardware and components. Programs and applications written in a low-level language are directly executable on the computing hardware without any interpretation or translation.
– Creates codes that is the fastest to execute.
Define low level languages?
– Low-level languages can refer to machine code, the binary instructions that a computer understands, or an assembly language that needs to be translated into machine code.
What are the pros of HLL?
– This means that programs written in a high-level language are easier to:
– read and understand as the language used is closer to human language
– write in a shorter time
– debug at the development stage
– maintain once in use
How is HLL designed?
– High-level languages are designed with programmers in mind; programming statements are easier to understand than those written in a low-level language.
Why is it useful for programmer to be expert in HLL?
– Once a programmer has learnt the techniques of programming in any HLL, these can be transferred to working in other high-level languages.
Give examples of high level languages?
– C++, Delphi, Java, Pascal, Python, Visual Basic are examples of high-level languages.
What is the use of high level languages?
– High-level languages enable a programmer to focus on the problem to be solved and require no knowledge of the hardware and instruction set of the computer that will use the program.
– High-level languages are designed to be used by the human operator or the programmer.
– Many high-level programming languages are portable and can be used on different types of computer.
Define high-level languages?
– A high-level language is any programming language that enables development of a program in a much more user-friendly programming context and is generally independent of the computer’s hardware architecture.
What is a program?
– A computer program is a list of instructions that enable a computer to perform a specific task. Like streaming videos, write reports, provide weather forecast and the provision of a multiplication tables tests.
– Computer programs can be written in high-level languages or low-level languages, depending on the task to be performed and the computer to be used.
Read more IGCSE Computer Science revision guides by Prodat here!
Tags:
algorithm design, the design and analysis of algorithm, examples of algorithm design, algorithm design course, design of algorithm, algorithm design definition, algorithm design in programming, algorithm design examples, algorithm design and analysis, algorithm design manual